MySQL 字符串函数 函数名称 函数功能说明 ASCII() 返回字符串 str 中最左边字符的 ASCII…
python:字符串string 开头r,b,u,f 含义, str bytes 转换 format, 字节转字符串
字符串开头r b u f各含义:
1、字符串前加 b
作用:
python3.x里默认的str是(py2.x里的)unicode, bytes是(py2.x)的str, b”“前缀代表的就是bytes
python2.x里, b前缀没什么具体意义, 只是为了兼容python3.x的这种写法
b'input\n' # bytes字节符,打印以b开头。 # 输出: # b'input\n'
2、字符串前加 r
例:r”\n\n\n\n\n\n”
作用:声明后面的字符串是普通字符串,相对的,特殊字符串中含有:转义字符 \n \t 什么什么的。
测试效果:
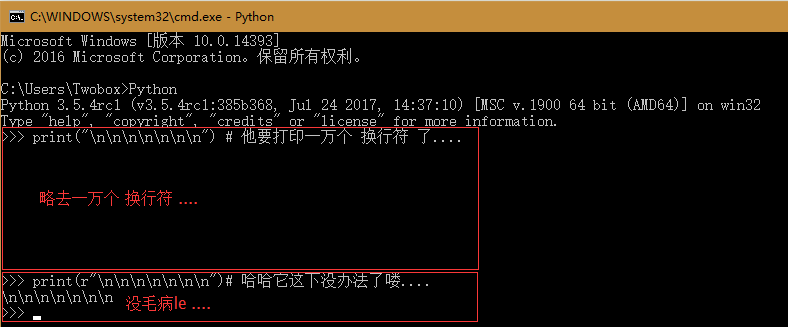
用途:一般用在 正则表达式、文件绝对地址、等等中。。。
r'input\n' # 非转义原生字符,经处理'\n'变成了'\\'和'n'。也就是\n表示的是两个字符,而不是换行。 # 输出: # 'input\\n'
3、字符串前加 u
例:u”我是含有中文字符组成的字符串。”
作用:后面字符串以 Unicode 格式 进行编码,一般用在中文字符串前面,防止因为源码储存格式问题,导致再次使用时出现乱码。
PS:不是仅仅是针对中文, 可以针对任何的字符串,代表是对字符串进行。一般英文字符在使用各种编码下,,基本都可以正常解析, 所以一般不带u。但是中文有事会出现问题,就要想以前在学校上机敲代码时候一样,优盘一插,源码一拷贝,一打开,中文部分全成框框乱码了。。。贼尴尬。。。
u'input\n' # unicode编码字符,python3默认字符串编码方式。 # 输出: # 'input\n'
4、字符串前加 f
Python提倡用一种,而且最好是只有一种方法来完成一件事。可是在字符串格式化方面Python好像没有做到这一点。除了基本的%-formatting
格式,还有str.format()
格式、以及string.Template
。根据对Python标准库的统计,目前string.Template
的使用屈指可数,str.format()
获得广泛使用,但是我们来与其他几种语言的字符串格式化对比一下:
已知name = 'Tom'
,我们如何打印出字符串'My name is Tom.'
?
Ruby:
puts 'My name is #{name}.'
JavaScript(ECMAScript 2015):
console.log(`My name is ${name}.`)
Python:
print('My name is {name}.'.format(name = name)) # 即便是简化的版本 print('My name is {}.'.format(name))
可以看出Python明显还不够简洁,于是,随着Python3.6版本在上周正式发布,Python又提供了一种字符串格式化语法——’f-strings’。
f-strings
要使用f-strings,只需在字符串前加上f
,语法格式如下:
f ' <text> { <expression> <optional !s, !r, or !a> <optional : format specifier> } <text> ... '
基本用法
>>> name = "Tom" >>> age = 3 >>> f"His name is {name}, he's {age} years old." >>> "His name is Tom, he's 3 years old."
支持表达式
# 数学运算 >>> f'He will be { age+1 } years old next year.' >>> 'He will be 4 years old next year.' # 对象操作 >>> spurs = {"Guard": "Parker", "Forward": "Duncan"} >>> f"The {len(spurs)} players are: {spurs['Guard']} the guard, and {spurs['Forward']} the forward." >>> 'The 2 players are: Parker the guard, and Duncan the forward.' >>> f'Numbers from 1-10 are {[_ for _ in range(1, 11)]}' >>> 'Numbers from 1-10 are [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]'
排版格式
>>> def show_players(): print(f"{'Position':^10}{'Name':^10}") for player in spurs: print(f"{player:^10}{spurs[player]:^10}") >>> show_players() Position Name Guard Parker Forward Duncan
数字操作
# 小数精度 >>> PI = 3.141592653 >>> f"Pi is {PI:.2f}" >>> 'Pi is 3.14' # 进制转换 >>> f'int: 31, hex: {31:x}, oct: {31:o}' 'int: 31, hex: 1f, oct: 37'
与原始字符串联合使用
>>> fr'hello\nworld' 'hello\\nworld'
注意事项
{}
内不能包含反斜杠\
f'His name is {\'Tom\'}' SyntaxError: f-string expression part cannot include a backslash # 而应该使用不同的引号,或使用三引号。 >>> f"His name is {'Tom'}" 'His name is Tom'
不能与'u'
联合使用
'u'
是为了与Python2.7兼容的,而Python2.7不会支持f-strings,因此与'u'
联合使用不会有任何效果。
如何插入大括号?
>>> f"{{ {10 * 8} }}" '{ 80 }' >>> f"{{ 10 * 8 }}" '{ 10 * 8 }'
与str.format()
的一点不同
使用str.format()
,非数字索引将自动转化为字符串,而f-strings则不会。
>>> "Guard is {spurs[Guard]}".format(spurs=spurs) 'Guard is Parker' >>> f"Guard is {spurs[Guard]}" Traceback (most recent call last): File "<pyshell#34>", line 1, in <module> f"Guard is {spurs[Guard]}" NameError: name 'Guard' is not defined >>> f"Guard is {spurs['Guard']}" 'Guard is Parker'
实例:
import time t0 = time.time() time.sleep(1) name = 'processing' print(f'{name} done in {time.time() - t0:.2f} s') # 以f开头表示在字符串内支持大括号内的python 表达式 #输出: processing done in 1.00 s
str与bytes转换:
'€20'.encode('utf-8') # b'\xe2\x82\xac20' b'\xe2\x82\xac20'.decode('utf-8') # '€20'
s1 = '123' print(s1) print(type(s1)) s2 = b'123' print(s2) print(type(s2)) 区别输出: 123 <class 'str'> b'123' <class 'bytes'>
Python 2 将字符串处理为 bytes 类型。
Python 3 将字符串处理为 unicode 类型。
#str转bytes: bytes('123', encoding='utf8') str.encode('123') #bytes转str: str(b'123', encoding='utf-8') bytes.decode(b'123')
本文:python:字符串string 开头r,b,u,f 含义, str bytes 转换 format, 字节转字符串